The 4 ES2022 Features You Should Know About
Updated: February 18, 2022•2 min read
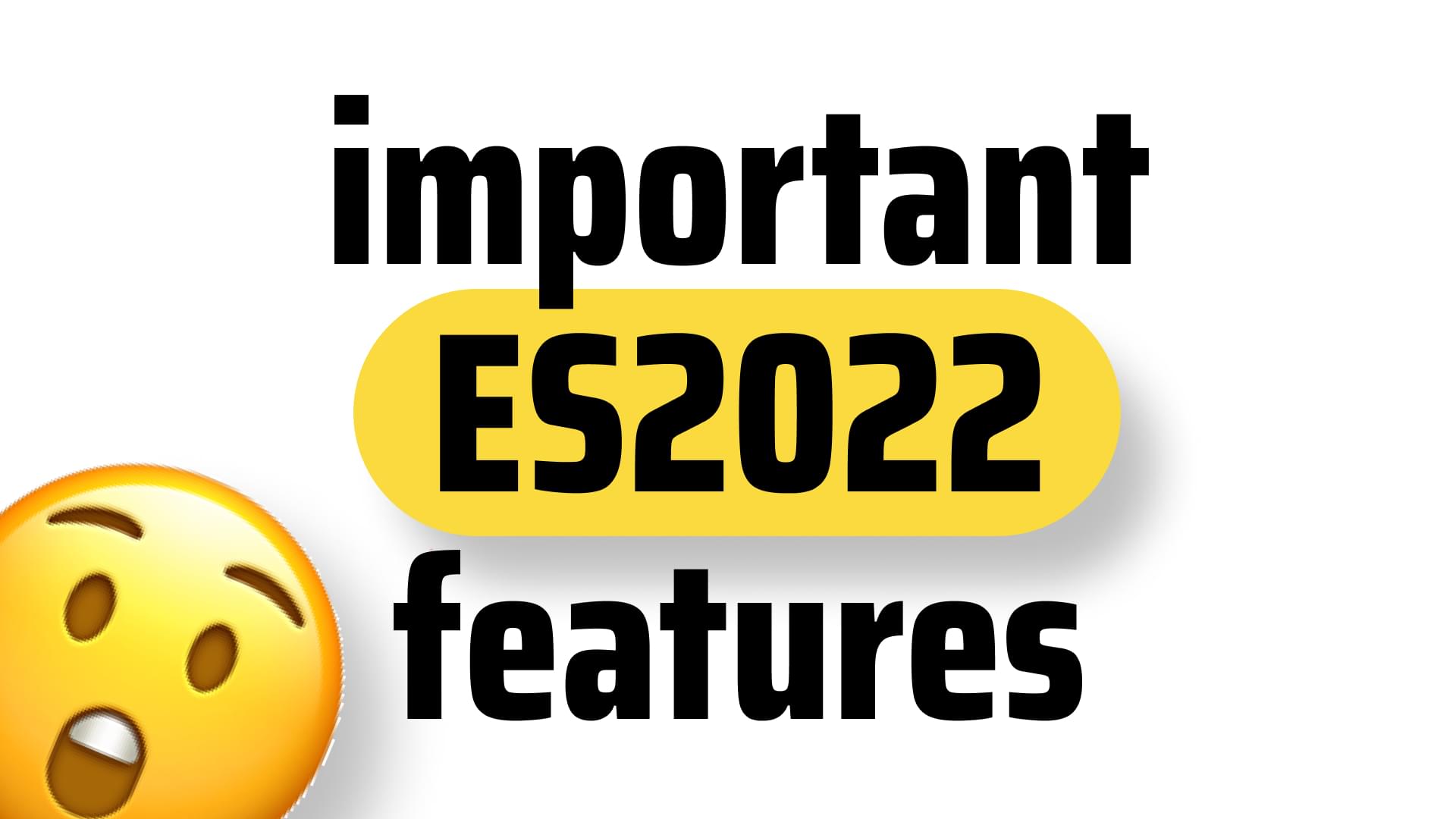
1. Method at() in arrays
Finally! ES2022 will give us a possibility to index array-like objects from the end. That’s a tiny feature, but it improves core readability when dealing with arrays
or strings
.
At()
method with positive number will work the same as indexing by []
, but with negative will allow accessing values from the end.
Instead of writing:
const arr = [1, 2, 3, 4];arr[arr.length - 2]; // 3arr.slice(-2)[0]; // 3const str = "1234";str[str.length - 2]; // '3'str.slice(-2)[0]; // '3'
We would be able to write:
const arr = [1, 2, 3, 4];arr.at(-2); // 3const str = "1234";str.at(-2); // '3'
2. Error cause
.cause
property on the error object would allow us to specify which error caused the other error.
try {doSomeComputationThatThrowAnError();} catch (error) {throw new Error("I am the result of another error", { cause: error });}
3. Top-level await
- It allows to load modules dynamically
const serviceName = await fetch("https://example.com/what-service-should-i-use");const service = await import(`/services/${serviceName}.js`);// ORconst params = new URLSearchParams(location.search);const theme = params.get("theme");const stylingFunctions = await import(`/styling-functions-${theme}.js`);
- It allows to conditionally render modules
const date = new Date();if (date.getFullYear() === 2023) {await require("/special-code-for-2023-year.js");}
4. Private slots and methods
They are private properties of the classes. ES2022 will give us the possibility to create them and get an error when we try to access them outside of the class. The same goes for private methods.
JS team chose to give them #
prefix.
Here is an example of private slots in action:
class Human {#name = "John";setName(name) {this.#name = name;}}const human = new Human();human.#name = "Amy"; // ERROR!human.setName("Amy"); // OK
And private method:
class Human {name = "John";constructor(name) {this.#setName("Amy"); // OK}#setName(name) {this.name = name;}}const human = new Human();human.#setName("Amy"); // ERROR!
Final notes
You can find all improvements here.
Don’t forget to clap 👏 in the comment section below if you learned something new